どうもこんにちは(こんばんは?おはようございます?)DICEです。
今回はどこを探してもC#で開発するDiscord Botでのスラッシュコマンドの登録方法が見当たらなかったので登録に成功した方法をまとめようと思います。
注意ポイント
Discord.netではスラッシュコマンドの受け側は開発段階のようなので、現状使用できません。
どうしても使いたい場合はdiscord.jsかdiscord.pyを使用するようにしましょう。
[st-card-ex url="https://www.dice-programming-etc.com/c-sharp-discord-questionary/" target="_blank" rel="nofollow" label="前回の記事" name="" bgcolor="red" color="" readmore=""]
そもそもスラッシュコマンドとは
簡単に言えば「/」を打った時に予測で出てくるコマンドのことです。
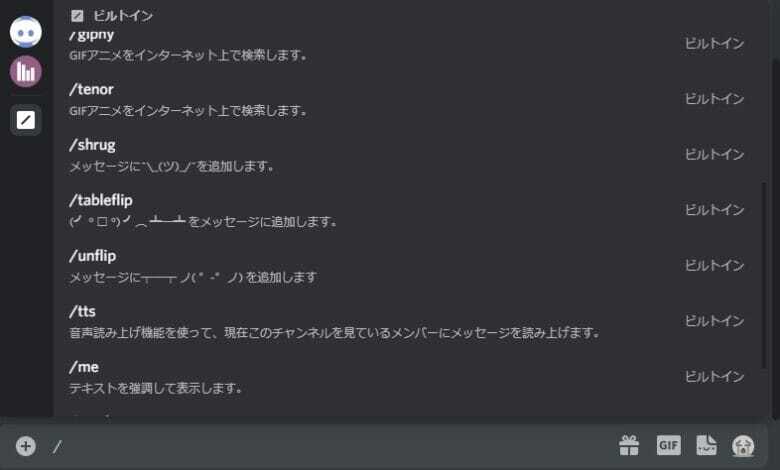
詳しくは以下のDiscord JP公式Twitterをご覧ください。
スラッシュコマンド:お気に入りのBotでこの機能が有効になったら、スラッシュボタン(/)を押すだけで、利用可能なすべてのBotコマンドのリストが呼び出せるよ pic.twitter.com/IUid66BNYR
— Discord Japan (@discord_jp) March 31, 2021
これを登録していきます。
Botにコマンドに関する権限を与える
Discord Developer Portalでボットに新しく権限を与える必要があります。
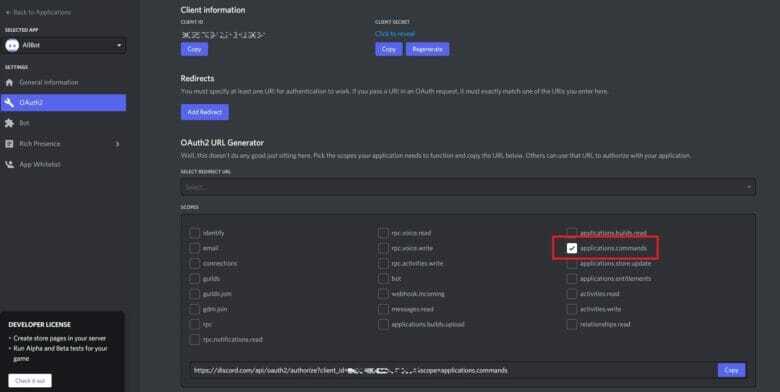
application.commandsにチェックを入れ、生成されたURLをブラウザで開きBotに権限を付与しましょう。
C#でスラッシュコマンドの登録
そもそもの登録方法についてですが、詳しいことはDiscordの公式開発ドキュメントを見たほうが良いかもしれません。
[st-card-ex url="https://discord.com/developers/docs/interactions/slash-commands" target="_blank" rel="nofollow" label="公式ドキュメント" name="" bgcolor="#6495ed" color="" readmore=""]
簡単に言うと特定のURLに対してコマンドの情報を含むJSON形式のデータをPOST送信することでスラッシュコマンドを登録することができます。
今回の開発ではHttpClientを使用していきます。
JSONについてですが、今回は簡単な感じで実装するのでDictionaryを使用します。
クラスの定義などは省略し、実装メソッドのみ書きます。
using System.Collections.Generic;
using System.Text;
using System.Net.Http;
using System.Threading.Tasks;
using Newtonsoft.Json;
using System.Collections;
//...
public async Task CommandRequests()
{
//-----------------------------------Jsonデータ---------------------------------
var json = new Dictionary<string, object>(){
{"name","permissions" },
{"description","Get or edit permissions for a user or a role" },
{"options",new ArrayList(){
new Dictionary<string, object>(){
{"name","user" },
{"description", "Get or edit permissions for a USER!" },
{"type",2 },
{"options",new ArrayList(){
new Dictionary<string, object>(){
{ "name","get" },
{"description","Get permissions for a USER!!" },
{"type",1 },
},
new Dictionary<string, object>(){
{ "name","edit" },
{"description","Edit permissions for a user" },
{"type",1 },
}
}
}
},
new Dictionary<string, object>(){
{"name","role" },
{"description", "Get or edit permissions for a role" },
{"type",2 },
{"options",new ArrayList(){
new Dictionary<string, object>(){
{ "name","get" },
{"description","Get permissions for a Role" },
{"type",3 },
},
new Dictionary<string, object>(){
{ "name","edit" },
{"description","Edit permissions for a role!" },
{"type",3 },
}
}
}
}
}
}
};
//----------------------------------------------------------------------------------
string url = "https://discord.com/api/v8/applications/<my_application_id>/commands";
//string url = "https://discord.com/api/v8/applications//<my_application_id>/guilds/<guild_id>/commands";
var client = new HttpClient();
try {
var content = JsonConvert.SerializeObject(json);
var builder = new UriBuilder(new Uri(url));
var request = new HttpRequestMessage(HttpMethod.Post, builder.Uri);
request.Headers.Add("Authorization", "Bot " + token);
request.Content = new StringContent(content, Encoding.UTF8, "application/json");
HttpResponseMessage response = await client.SendAsync(request);
}
catch(Exception e)
{
Console.WriteLine(e);
}
}
//...
13行目~55行目まででJSONを定義しています。
57行目のURLにPOST送信することでコマンドを登録するようです。
このURLで登録するとグローバルコマンドとなるのでコマンドの反映に約1時間かかります。
58行目はギルドコマンドなのでguild_idに指定したサーバーでのみ使用できるコマンドを登録できます。
こちらは即時反映されるようです。
61行目で定義したJSONを文字列化しています。
POST送信するURLが「https」なのでUriBuilderを使用します。
で、もろもろ設定してPOST送信をすると……
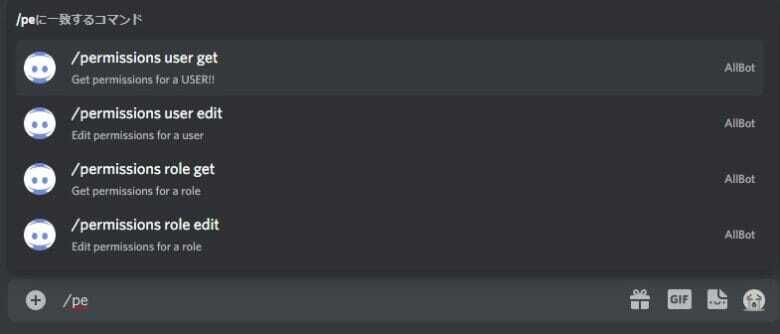
という感じで反映されます。
それだけです。
これを実装しただけではスラッシュコマンドは使用できません。
スラッシュコマンドを受け付ける側が必要です。
ただ、最初にも書きましたがDiscord.Netでは未だ開発中とのことで実装はできません。
まとめ
スラッシュコマンドの受け側を開発できないので記事としては以上になります。
少しでも参考になれば幸いです。
今後Discord.Netでもスラッシュコマンドの受け側が実装できるようになったら、受け側の実装をしていきますね。
それまではdiscord.jsかdiscord.pyを使用して受け側を実装していこうと思います。